CS307: Material and Lighting
Plan
- Recap. Surface materials, reflectance, lights
- ambient, diffuse, specular
- Lambert and Phong models
- Spotlights!
- Materials and light sources in Three.js
- Exercises: pink ball, golden treasure, add light bulb to Luxo
Camera Partners
- 2 Ailie+Claire T
- 4 Faith+Lindsay
- 6 Ari+UGyeong
- 8 Hae Rin+Ingrid
- 10 Anastacia+Martha
- 12 Ariana+Joyce
- 14 Emily+Heidi
- 16 Gabby+Mulan
- 18 Hana+Kate
- 20 Genevieve+Jenny
- 22 Amy+Claire M
- 24 Alo+Marilyn
- 26 Elisha+Rik
Quiz Questions
Let's look at the quiz questions.
Lights: Spotlights
We learned about
- ambient light
- directional light
- point lights
Now, let's turn to spotlights
All light-source classes are subclasses of the Light
class, which is a
subclass of Object3D
, which has a position
property that can be
set with position.set(x,y,z)
before adding the light source to the scene. For
a directional light, the combination of the position of the light and the position of
a target determine the direction of the light onto surfaces in the scene.
Spotlights have more parameters, and can also be directed toward a target location or object in the scene (the default target is the origin of the scene):
var spotLight = new THREE.SpotLight ( color, // rgb color of the source, default white intensity, // multiplication factor, default 1 distance, // distance where light ends, default 0 angle, // width of beam in radians, default Math.PI/2 penumbra, // % spotlight cone attenuated (0-1), default 0 decay // amount light decays with distance, default 1 ); spotLight.position.set(x,y,z); spotLight.target.position.set(xt,yt,zt); scene.add(spotLight); scene.add(spotLight.target);
If the desired target is a location other than the origin of the scene, the target (
spotLight.target
in this case) must be
added to the scene.
Let's explore this spotlight demo
The Three.js example: Three.js spotlight demo
Exercises: Pink Ball, Golden Treasure, and Luxo Lamp
Scott took these photos of his daughter's pink plastic ball:
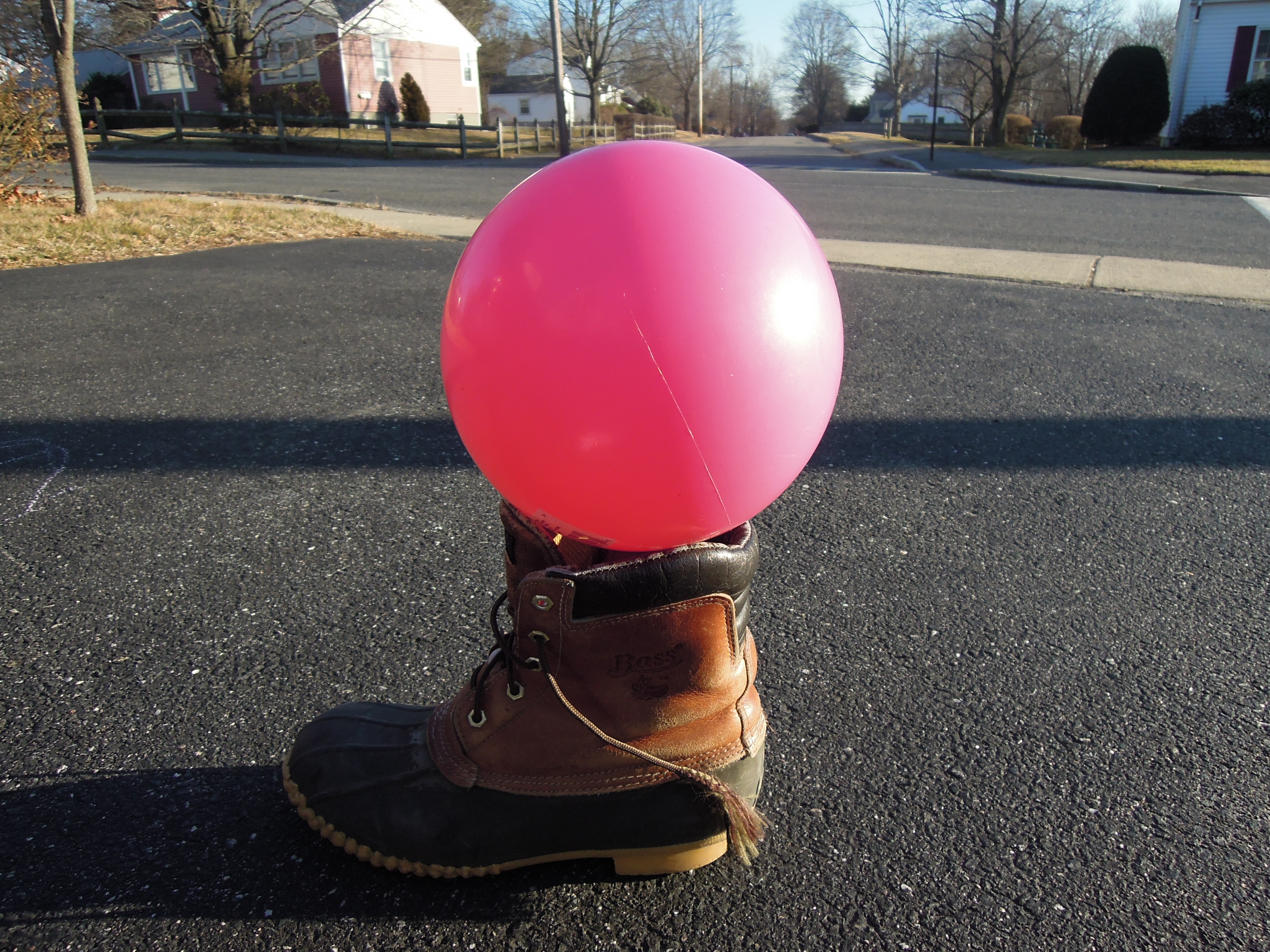
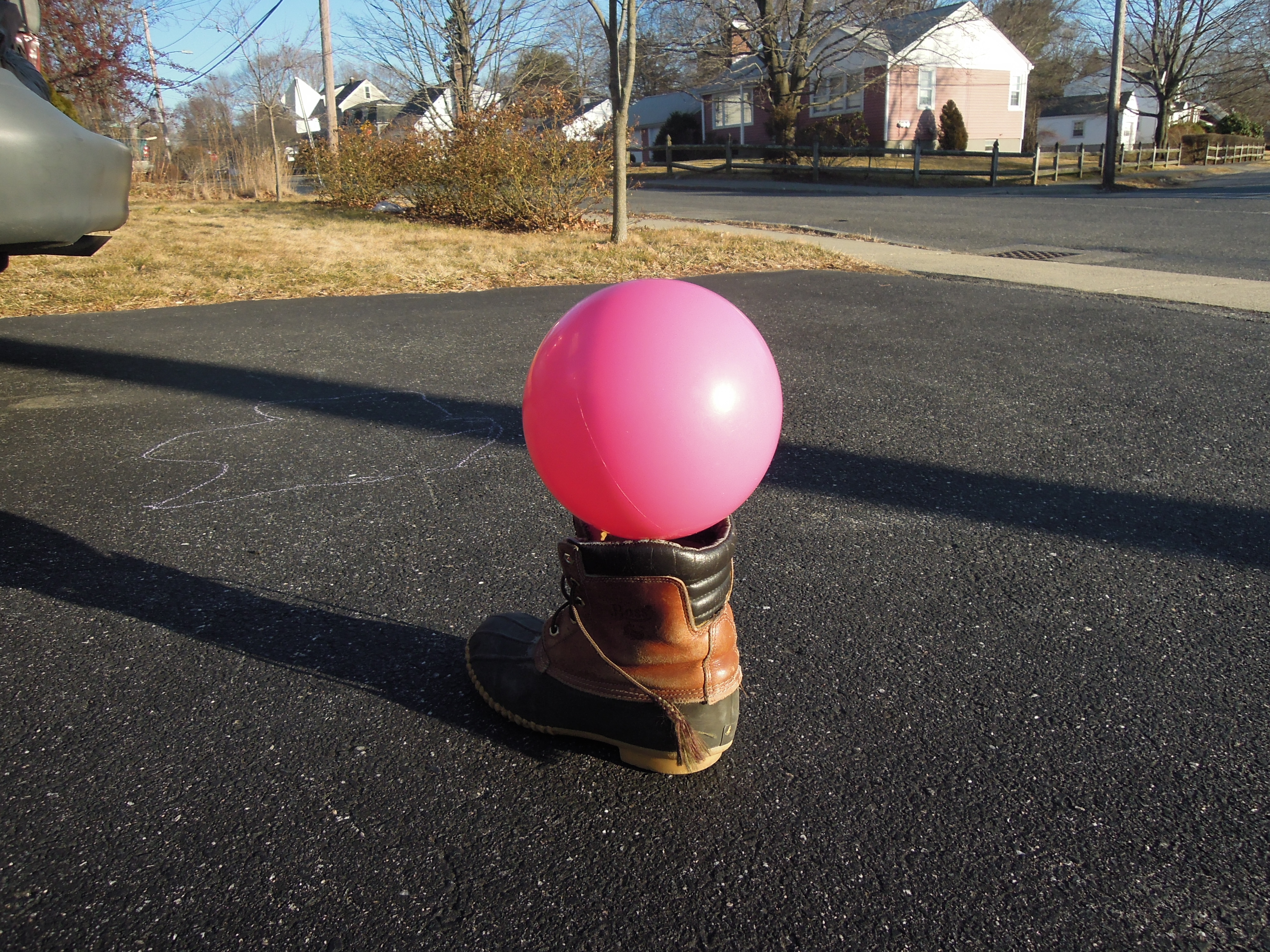
Do you see the effects of specularity? If so, where?
Do you see the effects of diffuse reflection? If so, where?
Do you see the effects of ambient light? If so, where?
Exercise: Imitate the pink ball
Using this starter code, try to imitate the pink plastic ball.
Here's a GUI to help: pink ball GUI
Your final result might look something like this:
Here is a possible code solution: pinkBall.html
Exercise: Golden treasure
Using this starter code that displays a scene of solid gold-colored objects, modify the code to use Phong material and light sources to make the objects look more like shiny golden treasure (see "before" and "after" pictures below — you can probably do better than me!)
Real Luxo lamps have bulbs that emit light, as in this scene with Luxo Mom and Junior:
Exercise: Add a light source to the Luxo lamp
Starting with this luxo-bulb-start.html code file, complete these steps to add a spotlight for each Luxo lamp in the scene:
- Modify the materials stored in the
colorMaterials
array to be Phong materials instead of basic materials - Fill in the inputs to the
THREE.SpotLight()
constructor in the functionaddLuxoBulb()
that adds a spotlight to the scene for a Luxo lamp. You can mostly use default values here, but think about whatangle
to use in this case, based on the dimensions of the actual cone, and experiment with thepenumbra
value - Modify the position of
bulbTarget
in theaddLuxoBulb()
function - the target should be on the ground, at a distancedist
from the origin of the lamp(posX,0,posZ)
and at an angleangleY
(these variables are all defined in the code), as shown in the diagrams below: - Add two calls to the
addLuxoBulb()
function, to add bulbs for Mom and Junior
Your final code might look something like this: luxo-bulb-solution.html
Useful Facts about Material and Lighting
- Spotlights (and lights in general), are not affected by the
transformations that we apply to geometry. Therefore, it doesn't work
to add a light to, say, a clown's hat, and add that to the head, which
gets added to the body, and so forth. You should add your lights
directly to the scene, and use
global
coordinates when parameterizing them. - Lights have a boolean attribute,
.visible
. If they are not visible, they don't contribute to the scene. This makes them easy to turn on and off. You don't have to re-build anything, though you will need to re-render. - Spotlights only interact properly with
THREE.MeshPhongMaterial
, not withTHREE.MeshLambertMaterial
. This is not what the Three.js documentation says.
Preparation for Next Time
Next week, we'll talk about texture mapping which can give a surface a more realistic, photo-like appearance and enable you to create your own visual texture on a surface. To prepare:
- Reading for next week: Texture Mapping